Great Software Requirement
- Great software always does what the customer wants it to. So even if customers think of new ways to use the software, it doesn’t break or give them unexpected results.
- Great software is code that is object-oriented. So there’s not a bunch of duplicate code, and each object pretty much controls its own behavior. It’s also easy to extend because design is solid and flexible.
- Great software use tried-and-true design patterns and principles. Objects are loosely coupled, and code is open for extension but closed for modification. This also helps make the code more reusable, so you don’t have to rework everything to use parts of your application over and over again.
Object Properties
- Objects should do what their names indicate. If an object is named Jet, it should probably
takeOff()
andland()
, but it shouldn’ttakeTicket()
—that’s the job of another object, and doesn’t belong in Jet. - Each object should represent a single concept. You don’t want objects serving double or triple duty.
- Unused properties are a dead giveaway. If you’ve got an object that is being used with no-value or null properties often, you’ve probably got an object doing more than one job.
Some principle which will help achieve this:-
- Encapsulation: It is breaking application into logical parts that have a clear boundary. This allows an object to hide its data and methods from other objects.
- Delegation: It is giving another object the responsibility of handling a particular task. One object should be responsible for only one task. Object related operation should be performed within the class. It helps applications stay loosely coupled. That means objects are independent of each other. Changes to one object don’t require to make a bunch of changes to other objects.
Good Use Case Requirement
A use case is a technique for capturing the potential requirements of a new system or software change. Each use case provides one or more scenarios that convey how the system should interact with the end user or another system to achieve a specific goal. Basic requirement for a good use case to get the job done are
- To make sure you have a good set of requirements, develop use cases for system. A use case details the steps that a system takes to make something happen. A use case has a single goal, but can have multiple paths to reach that goal.
- A good use case has a starting and stopping condition, an external initiator, and clear value to the user. A use case is started off by an external initiator, outside of the system. If the use case doesn’t help the customer achieve their goal, then the use case isn’t of much use.
- There should be at least one use case for each goal that system must accomplish. After use cases are complete, refine requirements.
- When things go wrong, system must have alternate paths (use case) to reach the system’s goals.
- Alternate paths can be steps that occur only some of the time, or provide completely different paths through parts of a use case.
A complete path through a use case, from the first step to the last, is called a Scenario. Most use cases have several different scenarios, but they always share the same user goal.
Analysis and Design
- Well designed software are easy to change and extend.
- Use object oriented principles like encapsulation and inheritance to make software flexible. Encapsulate what varies. Code to an interface rather than to an implementation.
- If a bad design isn’t flexible, then CHANGE IT! Never settle on bad design, even if it’s your bad design that has to change.
- Make sure each of classes is cohesive: each of classes should focus on doing ONE THING really well. Each class application should have only one reason to change. Classes are about behavior and functionality.
Architecture Design
- Architecture helps you turn all your diagrams, plans, and feature lists into a well-ordered application.
- The features in your system that are most important to the project are architecturally significant.
- Focus on features that are the essence of your system, that you’re unsure about the meaning of, or unclear about how to implement first.
- Everything you do in the architectural stages of a project should reduce the risks of your project failing. If you don’t need all the detail of a use case, writing a scenario detailing how your software could be used can help you gather requirements quickly.
- When you’re not sure what a feature is, you should ask the customer, and then try and generalize the answers you get into a good understanding of the feature.
- Use commonality analysis to build software solutions that are flexible.
Development Approaches
- Use case development takes a single use case in your system, and focuses on completing the code to implement that entire use case, including all of its scenarios, before moving on to anything else in the application.
- Feature driven development focuses on a single feature, and codes all the behavior of that feature, before moving on to anything else in the application.
- Test driven development writes test scenarios for a piece of functionality before writing the code for that functionality. Then you write software to pass all the tests. Good software development usually incorporates all of these development models at different stages of the development cycle.
Solving Big Problems
- Gather feature list : Listen to the customer, and figure out what they want to build. Put together a feature list, in language the customer understands. Features are usually big things that a system does.
- Draw high level use case diagrams : Create blueprints of the system using use case diagrams (and use cases). Use cases are detail-oriented; focused more on the big picture. It tells everything the system needs to do. Use case diagram should account for all the features in your system.
- Break the problem in smaller modules : Break application up into modules, and then decide on an order in which to tackle each of modules.
- Find requirements of each module : Figure out requirements for each module, and make sure those fit in with the big picture. Commonality (how system behaves with respect to known system) and variability (what system should not do with respect to known system) give points of comparison between a new system and things you already know about.
- Do module domain analysis : Figure out how use cases map to objects in app, and make sure customer is on the same page as you are.
- Make preliminary design : Fill in details about your objects, define relationships between the objects, and apply principles and patterns.
- Implement the system : Write code, test it, and make sure it works. Do this for each behavior, each feature, each use case, each problem, until you’re done
Feature lists are all about understanding what software is supposed to do. It reflect system’s functionality. Use case diagrams let you start thinking about how software will be used, without getting into a bunch of unnecessary details. Use cases are requirements for how people and things (actors) interact with your system, and features are requirements about things that your system must do.
OOAD Design Process
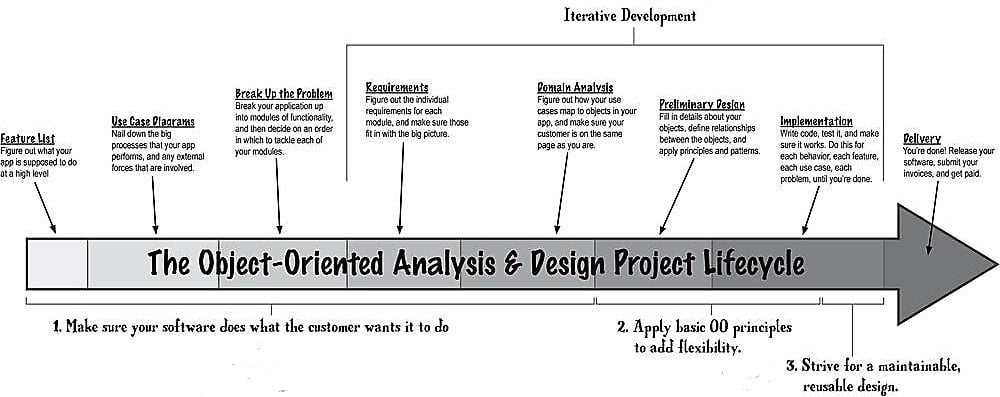